Programs on Arrays
1. WAP to print Array elements in reverse order.
2. WAP to copy elements of one array to another array.
3. WAP to merge two arrays.
4. WAP to search an element in an array.
5. WAP to print even and odd numbers in an array.
8. WAP to find the sum of all elements of an array.
9. WAP to find the sum of odd index elements of an array.
10. WAP to find the sum of even index elements of an array.
11. WAP to find the average of all elements of an array.
13. WAP to convert decimal(base 10) values to octal(base 8) values.
14. WAP to convert decimal(base 10) values to hexadecimal(base 16) values.
15. WAP to find the Largest Number in an Array.
16. WAP to print the smallest element in an array.
1. WAP to print Array elements in reverse order.
import java.util.Scanner;
public class ArrayElementsReverse {
public static void main(String[] args) {
int arr;
Scanner sc = new Scanner(System.in);
System.out.println("How many elements do you want in the array? ");
arr = sc.nextInt();
int[] elements = new int[arr];
for(int i=0;i<elements.length;i++) {
System.out.println("Enter element "+(i+1));
elements[i] = sc.nextInt();
}
for(int i = elements.length-1;i>=0;i--)
{
System.out.println("Reversed array: "+elements[i]);
}
}
}
Output:
How many elements do you want in the array?
2
Enter element 1
3
Enter element 2
2
Reversed array: 2
Reversed array: 3
2. WAP to copy elements of one array to another array.
import java.util.Scanner;
public class CopyElements {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter how many elements you want: ");
int n = sc.nextInt();
int[] elements = new int[n];
int[] elements1 = new int[n];
for(int i = 0; i<elements.length;i++) {
System.out.println("Enter the value of "+(i+1));
elements[i] = sc.nextInt();
}
for(int i = 0;i<elements.length;i++) {
elements1[i] = elements[i]; // assign array into another array
}
for(int i = 0;i<elements.length;i++) {
System.out.println("Original Array: "+elements[i]);
System.out.println("Copied Array: "+elements1[i]);
}
}
}
Output:
Enter how many elements you want:
2
Enter the value of 1
3
Enter the value of 2
5
Original Array: 3
Copied Array: 3
Original Array: 5
Copied Array: 5
3. WAP to merge two arrays.
import java.util.Scanner;
public class MergeTwoArrays {
public static void main(String[] args) {
int n1, n2;
Scanner sc = new Scanner(System.in);
System.out.println("How many elements do you want in 1st array?");
n1 = sc.nextInt();
int[] array1 = new int[n1];
for(int i = 0; i < array1.length; i++) {
System.out.println("Enter elements "+(i+1));
array1[i] = sc.nextInt();
}
System.out.println("How many elements do you want in 2nd array?");
n2 = sc.nextInt();
int[] array2 = new int[n2];
for(int i = 0; i < array2.length; i++) {
System.out.println("Enter elements "+(i+1));
array2[i] = sc.nextInt();
}
int total = array1.length + array2.length; // size of merge array
int[] array3 = new int[total];
for(int i = 0; i<array1.length;i++) {
array3[i] = array1[i]; // copied first element value into 3rd array
}
for(int i = 0; i<array2.length;i++) {
array3[i+array1.length] = array2[i];
}
System.out.println("The merged array is: ");
for(int i = 0; i<array3.length;i++) {
System.out.print(array3[i]+ " ");
}
}
}
Output:
How many elements do you want in 1st array?
2
Enter elements 1
1
Enter elements 2
4
How many elements do you want in 2nd array?
2
Enter elements 1
3
Enter elements 2
5
The merged array is:
1 4 3 5
4. WAP to search for elements in an array.
import java.util.Scanner;
public class SearchElmentsFromArray {
public static void main(String[] args) {
int n;
Scanner sc = new Scanner(System.in);
System.out.println("How many elements you want to search for: ");
n = sc.nextInt();
int[]arr=new int[n];
for(int i=0;i<n;i++) {
System.out.println("Enter the element you want to search for: ");
}
int value = sc.nextInt();
for(int i=0;i<n;i++)
{
if(arr[i]==value)
{
System.out.println("Element found!! ");
break;
}
else
System.out.println("Element not found!! ");
}
}
}
Output:
How many elements you want to search for:
1
Enter the element you want to search for:
4
Element not found!!
5. WAP to print even and odd numbers in an array.
public class EvenAndOddArray {
public static void main(String[] args) {
int[] array= {1,2,3,4};
System.out.println("Odd values: ");
for(int i = 0;i<array.length;i++) {
if(array[i] % 2 !=0 ) {
System.out.println(array[i]);
}
}
System.out.println("Even values: ");
for(int i = 0;i<array.length;i++) {
if(array[i] % 2 == 0 ) {
System.out.println(array[i]);
}
}
}
}
Output:
Odd values:
1
3
Even values:
2
4
6. WAP to find the number of elements in an array without using length.
import java.util.Scanner;
public class FindNumberOfElementsWithoutLength {
public static void main(String[] args) {
int count = 0;
int elements;
Scanner sc = new Scanner(System.in);
System.out.println("Enter how many elements you want in the array: ");
elements = sc.nextInt();
int[] arr = new int[elements];
for(int i = 0; i<elements;i++) {
System.out.println("Enter elements "+(i+1));
arr[i] = sc.nextInt();
count++;
}
System.out.println("Number of elements in array:"+count);
}
}
Output:
Enter how many elements you want in the array:
2
Enter elements 1
1
Enter elements 2
2
Number of elements in array:2
7. WAP to Print the kth Element in the Array (Accept kth value from user).
import java.util.Scanner;
public class KthElementInArray {
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
System.out.println("Enter how many elements you want in the array: ");
int n = sc.nextInt();
int[] elements = new int[n];
System.out.println("Enter all numbers you want in the array: ");
for(int i = 0; i< n;i++)
{
elements[i] = sc.nextInt();
}
System.out.println("Enter any number to find the kth element: ");
int num2 = sc.nextInt();
if(num2 <= elements.length)
System.out.println("The value is: "+elements[num2-1]);
}
}
Output:
Enter how many elements you want in the array:
4
Enter all numbers you want in the array:
1
5
6
7
Enter any number to find the kth element:
2
The value is: 5
8. WAP to find sum of all elements of an array.
import java.util.Scanner;
public class SumOfElements {
public static void main(String[] args) {
int num1, total = 0;
Scanner sc = new Scanner(System.in);
System.out.println("How many elements do you want in the array?");
num1 = sc.nextInt();
int[] array = new int[num1];
for(int i = 0; i<array.length;i++) {
System.out.println("Enter the value of "+(i+1));
array[i] = sc.nextInt();
total = total + array[i];
}
System.out.println("Sum of all elements in array: "+total);
}
}
Output:
How many elements do you want in the array?
2
Enter the value of 1
3
Enter the value of 2
2
Sum of all elements in array: 5
9. WAP to find sum of odd index elements of an array.
package assignments;
import java.util.Scanner;
public class OddElementsInArray {
public static void main(String[] args) {
int num1, total = 0;
Scanner sc = new Scanner(System.in);
System.out.println("How many elements do you want in the array?");
num1 = sc.nextInt();
int[] elements = new int[num1];
for(int i = 0; i<elements.length;i++) {
System.out.println("Enter the value of "+(i+1));
elements[i] = sc.nextInt();
}
for(int i = 0; i<num1;i++) {
if(i % 2 == 1) // odd position
{
total = total + elements[i];
}
}
System.out.println("Sum of value at odd position "+total);
}
}
Output:
How many elements do you want in the array?
3
Enter the value of 1
1
Enter the value of 2
4
Enter the value of 3
5
Sum of value at odd position 4
10. WAP to find sum of even index elements of an array.
package assignments;
import java.util.Scanner;
public class OddElementsInArray {
public static void main(String[] args) {
int num1, total = 0;
Scanner sc = new Scanner(System.in);
System.out.println("How many elements do you want in the array?");
num1 = sc.nextInt();
int[] elements = new int[num1];
for(int i = 0; i<elements.length;i++) {
System.out.println("Enter the value of "+(i+1));
elements[i] = sc.nextInt();
}
for(int i = 0; i<num1;i++) {
if(i % 2 != 1) // odd position
{
total = total + elements[i];
}
}
System.out.println("Sum of value at odd position "+total); }
}
Output:
How many elements do you want in the array?
3
Enter the value of 1
1
Enter the value of 2
4
Enter the value of 3
5
Sum of value at odd position 6
11. WAP to find the average of all elements of an array.
import java.util.Scanner;
public class AverageOfAllElementsInArray {
public static void main(String[] args) {
int num1, total = 0, average = 0;
Scanner sc = new Scanner(System.in);
System.out.println("How many elements do you want in the array?");
num1 = sc.nextInt();
int[] array = new int[num1];
for(int i = 0; i<array.length;i++) {
System.out.println("Enter the value of "+(i+1));
array[i] = sc.nextInt();
total = total + array[i];
}
average = total/array.length;
System.out.println("Average of all elements in array: "+average);
}
}
Output:
How many elements do you want in the array?
2
Enter the value of 1
10
Enter the value of 2
10
Average of all elements in array: 10
12. WAP to convert decimal(bas 10) values to binary(base 2) values.
int n,r,i=1,j;
int bin[] = new int[100];
Scanner sc = new Scanner(System.in);
System.out.println("Enter a number: ");
n = sc.nextInt();
while(n !=0)
{
bin[i]=n % 2; // remainder
i++;
n = n/2; // binary equivalent
//int divide by int = int
}
System.out.print("Binary number is: ");
for(j=i-1;j>0;j--)
{
System.out.print(bin[j]+" ");
}
System.out.print("\n");
}
}
Output:
Enter a number:
9
Binary number is: 1 0 0 1
13. WAP to convert decimal(bas 10) values to octal(base 8) values.
int n,r,i=1,j;
int bin[] = new int[100];
Scanner sc = new Scanner(System.in);
System.out.println("Enter a number: ");
n = sc.nextInt();
while(n !=0)
{
bin[i]=n % 8; // remainder
i++;
n = n/8; // binary equivalent
//int divide by int = int
}
System.out.print("Octal number is: ");
for(j=i-1;j>0;j--)
{
System.out.print(bin[j]+" ");
}
System.out.print("\n");
}
}
Output:
Enter a number:
20
Octal number is: 2 4
14. WAP to convert decimal(bas 10) values to hexadecimal(base 16) values.
int n,r,i=1,j;
int bin[] = new int[100];
Scanner sc = new Scanner(System.in);
System.out.println("Enter a number: ");
n = sc.nextInt();
while(n !=0)
{
bin[i]=n % 16; // remainder
i++;
n = n/16; // binary equivalent
//int divide by int = int
}
System.out.print("Hexadecimal number is: ");
for(j=i-1;j>0;j--)
{
System.out.print(bin[j]+" ");
}
System.out.print("\n");
}
}
Output:
Enter a number:
30
Hexadecimal number is: 1 14
15. WAP to find the Largest Number in an Array.
package assignments;
import java.util.Scanner;
public class LargestNumberFromArray {
public static void main(String[] args) {
int n1;
Scanner sc = new Scanner(System.in);
System.out.println("How many elements do you want in the array?");
n1 = sc.nextInt();
int[] array = new int[n1];
int max = array[0];
for(int i = 0; i < array.length; i++) {
System.out.println("Enter elements "+(i+1));
array[i] = sc.nextInt();
if(array[i] > max)
max = array[i];
}
System.out.println("Largest number in the array: "+max);
}
}
Output:
How many elements do you want in the array?
3
Enter elements 1
10
Enter elements 2
5
Enter elements 3
20
Largest number in the array: 20
16. WAP to print the smallest element in an array.
package assignments;
import java.util.Scanner;
public class SmallestNumberFromArray {
public static void main(String[] args) {
int n1;
Scanner sc = new Scanner(System.in);
System.out.println("How many elements do you want in the array?");
n1 = sc.nextInt();
int[] array = new int[n1];
int min = array[0];
System.out.println("Smallest number in the array: "+ min);
for(int i = 0; i < array.length; i++) {
System.out.println("Enter elements "+(i+1));
array[i] = sc.nextInt();
if(array[i] < min)
min = array[i];
}
}
Output:
How many elements do you want in the array?
3
Enter elements 1
1
Smallest number in the array: 1
Enter elements 2
2
Smallest number in the array: 1
Enter elements 3
4
Smallest number in the array: 1
17. WAP to print the sort element in an array using Bubble Sort.
2D Arrays in Java
1)Transpose of Matrix in Java
2. WAP to perform matrix addition. Accept matrices from user.
public static void main(String[] args) {
int r,c;
Scanner sc =new Scanner(System.in);
System.out.println("Enter no of Rows for Matrix 1: ");
r=sc.nextInt();
System.out.println("Enter no of Columns for Matrix 1: ");
c=sc.nextInt();
int[][] a = new int[r][c];
System.out.println("Enter elements of matrix a: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
a[i][j]=sc.nextInt();
}
}
System.out.println("Original Matrix Format Is: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
System.out.print(a[i][j]+ "\t");
}
System.out.println();
}
System.out.println("Enter no of Rows for Matrix 2: ");
r=sc.nextInt();
System.out.println("Enter no of Columns: ");
c=sc.nextInt();
int[][] b = new int[r][c];
System.out.println("Enter elements of matrix a for Matrix 2: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
b[i][j]=sc.nextInt();
}
}
System.out.println("Original Matrix Format Is: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
System.out.print(b[i][j]+ "\t");
}
System.out.println();
}
System.out.println("New Added Matrix Is: ");
int arr[][] = new int[r][c];
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
arr[i][j] = a[i][j] + b[i][j];
System.out.print(arr[i][j]+" ");
}
System.out.println();
}
}
}
Output:
Enter no of Rows for Matrix 1:
2
Enter no of Columns for Matrix 1:
2
Enter elements of matrix a:
1
2
3
4
Original Matrix Format Is:
1 2
3 4
Enter no of Rows for Matrix 2:
2
Enter no of Columns:
2
Enter elements of matrix a for Matrix 2:
5
6
7
8
Original Matrix Format Is:
5 6
7 8
New Added Matrix Is:
6 8
10 12
3. WAP to perform matrix subtraction. Accept matrices from user.
public static void main(String[] args) {
int r,c;
Scanner sc =new Scanner(System.in);
System.out.println("Enter no of Rows for Matrix 1: ");
r=sc.nextInt();
System.out.println("Enter no of Columns for Matrix 1: ");
c=sc.nextInt();
int[][] a = new int[r][c];
System.out.println("Enter elements of matrix a: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
a[i][j]=sc.nextInt();
}
}
System.out.println("Original Matrix Format Is: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
System.out.print(a[i][j]+ "\t");
}
System.out.println();
}
System.out.println("Enter no of Rows for Matrix 2: ");
r=sc.nextInt();
System.out.println("Enter no of Columns: ");
c=sc.nextInt();
int[][] b = new int[r][c];
System.out.println("Enter elements of matrix a for Matrix 2: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
b[i][j]=sc.nextInt();
}
}
System.out.println("Original Matrix Format Is: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
System.out.print(b[i][j]+ "\t");
}
System.out.println();
}
System.out.println("New Subtracted Matrix Is: ");
int arr[][] = new int[r][c];
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
arr[i][j] = a[i][j] - b[i][j];
System.out.print(arr[i][j]+" ");
}
System.out.println();
}
}
}
Output:
Enter no of Rows for Matrix 1:
2
Enter no of Columns for Matrix 1:
2
Enter elements of matrix a:
5
6
7
8
Original Matrix Format Is:
5 6
7 8
Enter no of Rows for Matrix 2:
2
Enter no of Columns:
2
Enter elements of matrix a for Matrix 2:
1
2
3
4
Original Matrix Format Is:
1 2
3 4
New Subtracted Matrix Is:
4 4
4 4
4. WAP to perform matrix multiplication. Accept matrices from user.
public static void main(String[] args) {
int r,c;
Scanner sc =new Scanner(System.in);
System.out.println("Enter no of Rows for Matrix 1: ");
r=sc.nextInt();
System.out.println("Enter no of Columns for Matrix 1: ");
c=sc.nextInt();
int[][] a = new int[r][c];
System.out.println("Enter elements of matrix a: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
a[i][j]=sc.nextInt();
}
}
System.out.println("Original Matrix Format Is: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
System.out.print(a[i][j]+ "\t");
}
System.out.println();
}
System.out.println("Enter no of Rows for Matrix 2: ");
r=sc.nextInt();
System.out.println("Enter no of Columns: ");
c=sc.nextInt();
int[][] b = new int[r][c];
System.out.println("Enter elements of matrix a for Matrix 2: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
b[i][j]=sc.nextInt();
}
}
System.out.println("Original Matrix Format Is: ");
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
System.out.print(b[i][j]+ "\t");
}
System.out.println();
}
System.out.println("New Multiplied Matrix Is: ");
int arr[][] = new int[r][c];
for(int i=0;i<r;i++) {
for(int j=0;j<c;j++) {
arr[i][j]=0;
for(int k=0;k<r;k++) {
arr[i][j] = a[i][k] + b[k][j];
System.out.print(arr[i][j]+" ");
}
System.out.println();
}
}
}
}
Output:
Enter no of Rows for Matrix 1:
2
Enter no of Columns for Matrix 1:
2
Enter elements of matrix a:
1
2
3
4
Original Matrix Format Is:
1 2
3 4
Enter no of Rows for Matrix 2:
2
Enter no of Columns:
2
Enter elements of matrix a for Matrix 2:
1
2
3
4
Original Matrix Format Is:
1 2
3 4
New Added Matrix Is:
2 5
3 6
4 7
5 8
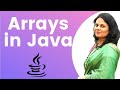
No comments: